- September 9, 2019
- Posted by: Vikas Chowdhury
- Categories:
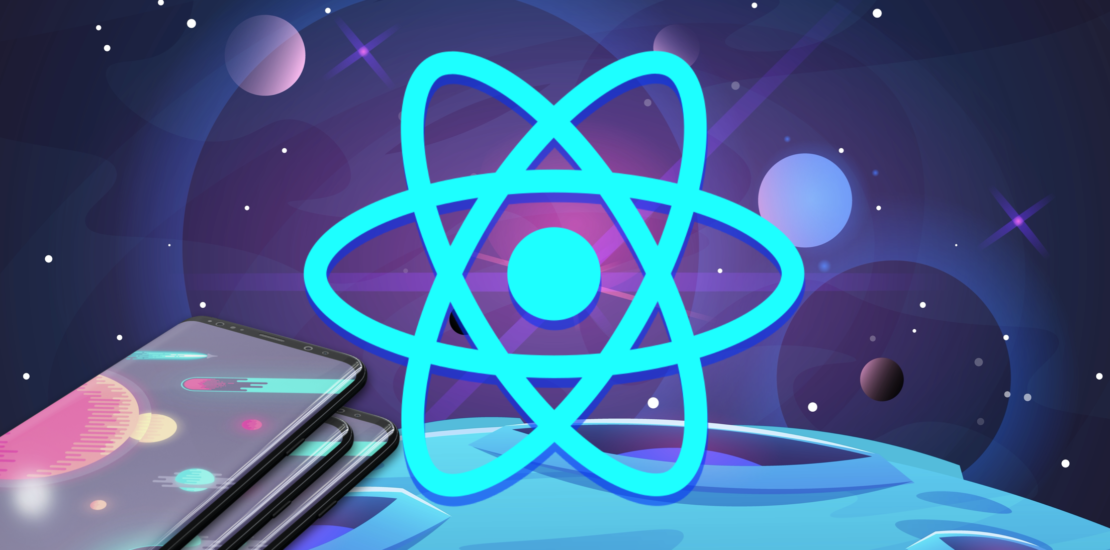
There are many cases where providing a url to transition a user to a specific point in your app on load makes a lot of sense.For Instance, password reset functionality requires external verification from email and mobile SMS.With Deep linking feature we can redirect user from verified link to Password Reset screen on App.
Linking will provide for us an API that will allow us to listen for an incoming linked url, and then we can handle the url like
componentDidMount() {
Linking.addEventListener(‘url’, this.handleOpenURL);
}
componentWillUnmount() {
Linking.removeEventListener(‘url’, this.handleOpenURL);
}
handleOpenURL(event) {
console.log(event.url);
const route = e.url.replace(/.*?:\/\//g, ”);
// do something with the url, in our case navigate(route)
}
When a user in an external app or website clicks on one of our links, we will open in our new app and navigate to the intended url:
yournxtapp://ResetPassword/0
yournxtapp://ResetPassword/1
Above will navigate to the ResetPassword route, and then show a profile based on the id.
To start with, we need to add following in your page where linking is desired.
For e.g. ResetPassword component we can add linking as following
import React from ‘react’;
import { Platform, Text, Linking } from ‘react-native’;
export default class ResetPassword extends React.Component {
static navigationOptions = { // A
title: Reset Password,
};
componentDidMount() { // B
if (Platform.OS === ‘android’) {
Linking.getInitialURL().then(url => {
this.navigate(url);
});
} else {
Linking.addEventListener(‘url’, this.handleOpenURL);
}
}
componentWillUnmount() { // C
Linking.removeEventListener(‘url’, this.handleOpenURL);
}
handleOpenURL = (event) => { // D
this.navigate(event.url);
}
navigate = (url) => { // E
const { navigate } = this.props.navigation;
const route = url.replace(/.*?:\/\//g, ”);
const id = route.match(/\/([^\/]+)\/?$/)[1];
const routeName = route.split(‘/’)[0];
if (routeName === ‘ResetPassword’) {
navigate(Profile, { id, name: vikas })
};
}
render() {
return <Text>Reset your password!</Text>;
}
}
- We declare a title using static navigationOptions for react-navigation to show a title when we are on this route.
- If we are on Android, we immediately call the navigate method passing in the url. If we are on iOS, We add an event listener to call handleOpenUrl when an incoming link is detected.
- We delete the Linking listener on componentWillUnmount
- When handleOpenURL is called, we pass the event url to the navigate method.