- January 9, 2021
- Posted by: Romil Lodaya
- Categories:
As the title suggests, how we can show notification count on Android badge? Our initial goal was to show the badge count of unread messages. But first, we searched for 3rd party library which solves our purpose. And we tried react-native-android-badge. But it was not working as per our expectations. So finally, we decide to try Android Native Modules.
To Know How to setup and create Android Native Modules, Please visit the link for detailed documentation – [https://reactnative.dev/docs/native-modules-android]
To Create a Badge Native module you need to create two files. Let’s called it BadgeModule.java and BadgePackage.java inside the folder android->app->src->main->java->com->[PROJECT_NAME].
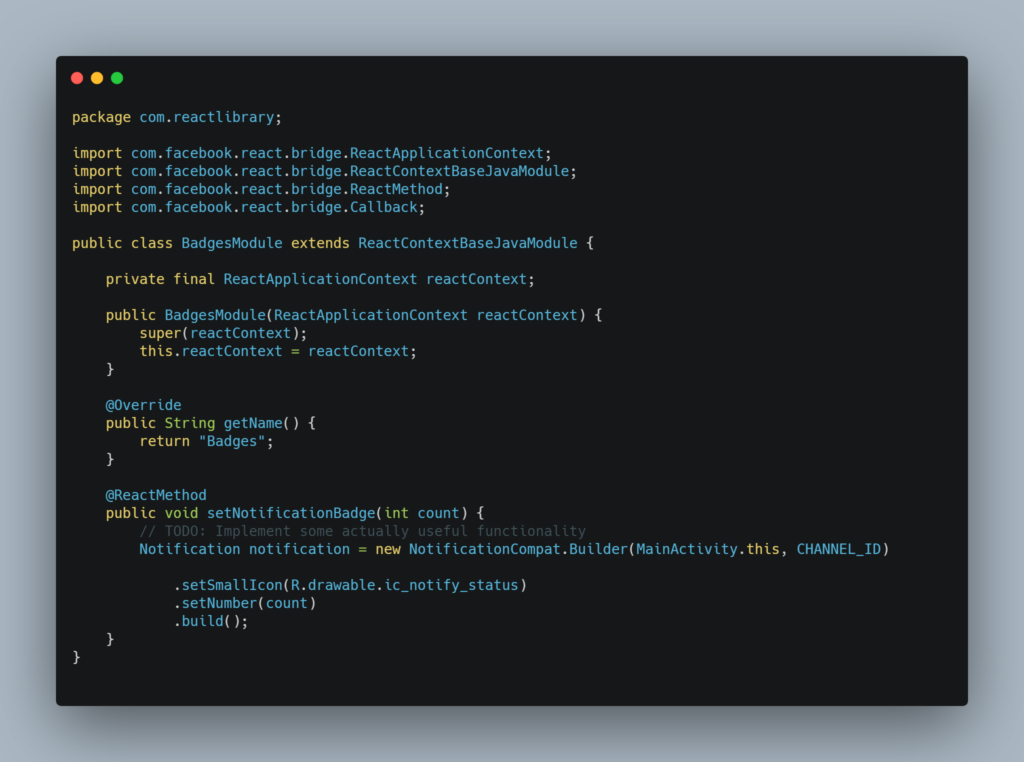
As you can see, BadgesModule class extends the ReactContextBaseJavaModule class. For Android, Java native modules are written as classes that extend ReactContextBaseJavaModule and implement the functionality required by JavaScript.
Module Name:
All Java native modules in Android need to implement the getName() method. This method returns a string, which represents the name of the native module. The native module can then be accessed in JavaScript using its name. For example, in the below code snippet, getName() returns “BadgesModule”.
Export a Native Method to JavaScript:
Next, you will need to add a method to your native module that will create calendar events and can be invoked in JavaScript. All native module methods meant to be invoked from JavaScript must be annotated with @ReactMethod.
Set up a method setNotificationBadge() for BadgesModule that can be invoked in JS through BadgesModule.setNotificationBadge(). For now, the method will take in a count as argument.
Android uses the NotificationCompat builder method with CHANNEL_ID and activity as an argument. Along with this, you can add more options like .setSmallIcon(), .setNumber(). Pass count variable to .setNumber(count) which will update badge count on Application Icon.
BadgePackage.java file is used for importing the BadgeModule file as a NativeModule list.
To use the native module in JS file first you need to first create a js file e.g. Badge.js and import NativeModules from ReactNative and then after that import Native Module name. In our case,
module.exports = NativeModules.Badges;
Now the final step is to use the actual file where you want to update the badge count. First import Badge.js file after that call setNotificationBadge method with count as an argument.
e.g. Badges.setNotificationBadge(2);
Checkout complete example for ref: https://github.com/theromie/RN-Badges