- December 12, 2023
- Posted by: Vikas Chowdhury
- Category: Programming
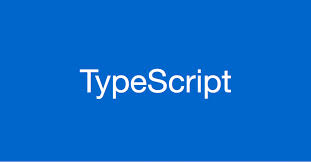
TypeScript is a superset of JavaScript which primarily provides optional static typing, classes and interfaces.
Typescript was not designed to supersede or replace Javascript. In fact, it never overrides existing behavior. It takes the existing behaviors of Javascript to correct its limitations and leverage common issues with the language.
One of the benefits of TypeScript is better tooling. TypeScript enables IDEs to provide a richer environment for spotting common errors as you type the code
There are many differences between Typescript and Javascript. Here are just a few:
- TypesScript is an Object oriented programming language whereas JavaScript is a scripting language (with support of OOPS features).
- TypeScript has static typing whereas JavaScript does not.
- TypeScript uses types and interfaces to describe how data is being used.
- TypeScript has interfaces which are a powerful way to define contracts within your code.
- TypeScript supports optional parameters for functions where JavaScript does not.
Since JavaScript supports classes and object-oriented programming, so does TypeScript. You can use an interface declaration with classes:
interface YNEmployee {
name: string;
id: number;
}
class YNEmployeeDetails {
name: string;
id: number;
constructor(name: string, id: number) {
this.name = name;
this.id = id;
}
}
const user: YNEmployee = new YNEmployeeDetails(“Vikaas”, 4);
With TypeScript, you can create complex types by combining simple ones.
type YNEmployeeDepartment = “accounts” | “hr” | “delivery”;
Generics
Generics provide variables to types. A common example is an array. An array without generics could contain anything. An array with generics can describe the values that the array contains.
type StringArr = Array<string>;
type NumberArr = Array<number>;
type ObjectWithNameArr = Array<{ name: string }>;
You can declare your own types that use generics:
interface YourNXT<Type> {
add: (obj: Type) => void;
get: () => Type;
}
// This line is a shortcut to tell TypeScript there is a
// constant called `YourNXT`, and to not worry about where it came from.
declare const backpack: YourNXT<string>;
Installation
If you already have installed Node Package manager, you can simply install TypeScript with following command
npm install -g typescript
noImplicitAny
According to the documentation, the definition of noImplicitAny is to “raise errors on expressions and declarations with any implied any type.”
This means that whenever TypeScript can infer a type, you will get an error if you allow noImplicitAny. This example can be seen by passing function arguments.
function print(argument) {
send(argument);
}
print(“hello”);
print(4);
In the above code, what are valid arguments for the print function? If you don’t add a type to the function argument, TypeScript will assign the argument of type any, which will turn off type checking.